Typescript in UI Editor
What is TypeScript Used For?
TypeScript extends JavaScript and enhances the developer experience.
It helps software developers adding type safety to their projects and provides features such as type aliases, interfaces, abstract classes, encapsulation, inheritance, and function overloading.
Overall, TypeScript makes it easier to create large and complex projects and compiles to JavaScript.
If you need more information about TypeScript, you can check out the resources below:
Where Can You Use Typescript In Studio UI Editor?
You can use typscript in component events. Which will reduce the likelihood of runtime errors in production and allow you to reorganize code more securely in large-scale applications.
How To Access The Quick Library?
The Quick Library is located under the quick object. Along with the quick library, you can quickly access reusable code fragments, thereby speeding up the development process
Intellisense preview | Example (EM.trace) |
---|---|
![]() | ![]() |
Important usages of the Quick Library
Data Storage & Data Transfer Between Pages with Store
quick.store.set(name: string, value: any);
quick.store.set('personalInfo', storeData);
quick.store.get(name: string);
quick.store.get(quick.store.get('$personalInfo').name);
quick.store.delete(name: string);
quick.store.delete('&personalInfo')
quick.store.delete('$personalInfo')
quick.store.delete('#personalInfo')
Transition Between Pages
quick.Quick.go("<<qjson:6h0uw50w-dd8q-xlq5-aoj2-ugtwkel1>>")
How To Access Components in TypeScript?
Components are located under the Components object.
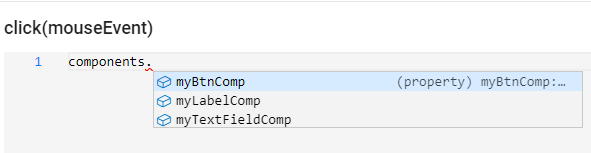
- You can directly set the value of a component.
Example:
components.UserNameComp.value = "Orbay";
- You can get and set the value of a component through an object.
Example:
interface IUser {
id: number;
name: string;
}
const myUser: IUser = {
id: 1,
name: "Orbay"
};
components.UserNameComp.value = myUser.name;
Return From a Function in Event
In the following example; It uses the filter method to create a new array with only the elements that match the given search value, and then returns the length of that filtered array.
function count(items:Array<number>, search){ //The count function takes two parameters
const result = items.filter(item => item == search).length; //Inside the function, it creates a new variable result and assigns it the length of the filtered array.
//The filter method is used to create a new array with only the elements that match the given search value
return result; // return of a function
}
const items = [1,2,3,4,5,4];
const retVal = count(items, 4); //we call the counting function with an array [1, 2, 3, 4, 5, 4] and search for the value of 4. The returned value is stored in the retVal variable.
quick.EM.trace("result: " + retVal);
quick.return(retVal); // return of an event (stop execution and return)
quick.EM.trace("this line will not execute");//will not be executed because it comes after the return statement.